Table of Contents
What Are HTML Tables?
HTML tables are essential elements for organizing and displaying structured data on web pages. Using a grid system of rows and columns, tables help present information like:
- Class schedules
- Product comparisons
- Statistical data
- Pricing charts
Table Anatomy 101
Every HTML table consists of these core components:
HTML
<table>
<tr> <!-- Table Row -->
<th>Header</th> <!-- Header Cell -->
<td>Data</td> <!-- Standard Cell -->
</tr>
</table>
Basic Table Example
HTML
<table>
<tr>
<th>Student</th>
<th>Grade</th>
</tr>
<tr>
<td>Alice</td>
<td>A+</td>
</tr>
</table>
Output Result
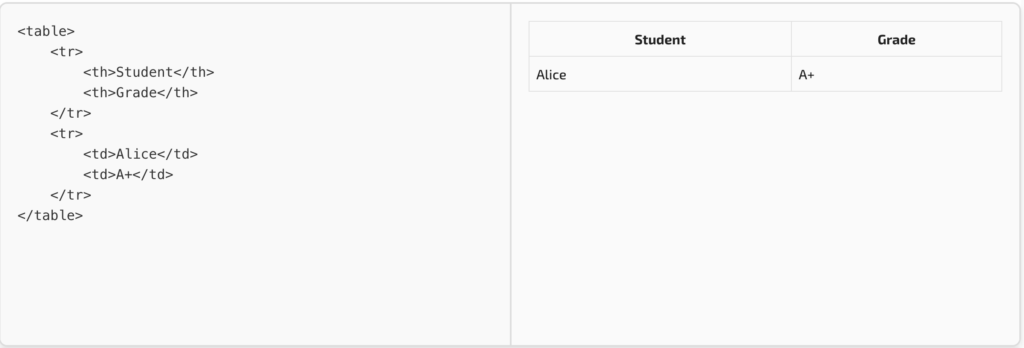
Enhancing Table Appearance
Modern styling with CSS (recommended):
CSS
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
border: 1px solid #ddd;
padding: 12px;
text-align: left;
}
th {
background-color: #f8f9fa;
}
</style>
Advanced Table Features
Cell Merging Techniques
Column spanning example:
HTML
<tr>
<th colspan="2">Annual Report</th>
</tr>
Accessibility Best Practices
HTML
<table aria-describedby="table-desc">
<caption>Monthly Sales Data</caption>
<thead>
<tr>
<th scope="col">Month</th>
<th scope="col">Sales</th>
</tr>
</thead>
<tbody>
<!-- Data rows -->
</tbody>
</table>
Responsive Table Design
HTML
<div style="overflow-x: auto;">
<table>
<!-- Wide table content -->
</table>
</div>
Common Pitfalls to Avoid
- ❌ Using tables for page layout
- ❌ Creating overly complex nested tables
- ❌ Forgetting accessibility features
- ❌ Using deprecated attributes like cell padding
Complete Table Example
HTML
<div style="overflow-x: auto;">
<table>
<caption>Class Schedule</caption>
<thead>
<tr>
<th scope="col">Time</th>
<th scope="col" colspan="2">Monday</th>
</tr>
</thead>
<tbody>
<tr>
<td>9:00 AM</td>
<td>Mathematics</td>
<td rowspan="2">Lab</td>
</tr>
</tbody>
</table>
</div>
Output Results

Read Other Tutorials
- HTML Tags: Mastering Headings, Paragraphs, and Links for Beginners
- What is HTML? Understanding the Basic Structure for Beginners
- HTML Forms: A Comprehensive Guide for Beginners
- HTML Lists Tutorial: Organize Content Effectively
- HTML Images Tutorial: A Beginner’s Guide to Adding and Optimizing Images
Complete Code for Example of HTML Tables
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML Table Example - Student Class Schedule</title>
<style>
/* Modern Table Styling */
.schedule-table {
border-collapse: collapse;
width: 100%;
margin: 25px 0;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
box-shadow: 0 0 20px rgba(0, 0, 0, 0.15);
}
.schedule-table caption {
font-size: 1.5em;
margin: 0.5em 0;
font-weight: bold;
color: #2c3e50;
}
.schedule-table thead tr {
background-color: #3498db;
color: #ffffff;
text-align: left;
}
.schedule-table th,
.schedule-table td {
padding: 12px 15px;
}
.schedule-table tbody tr {
border-bottom: 1px solid #dddddd;
}
.schedule-table tbody tr:nth-of-type(even) {
background-color: #f8f9fa;
}
.schedule-table tbody tr:last-of-type {
border-bottom: 2px solid #3498db;
}
.schedule-table tbody td[colspan] {
background-color: #ecf0f1;
font-weight: bold;
}
.responsive-table {
overflow-x: auto;
margin: 20px 0;
}
</style>
</head>
<body>
<div class="responsive-table">
<table class="schedule-table">
<caption>Computer Science Class Schedule</caption>
<thead>
<tr>
<th scope="col">Time</th>
<th scope="col" colspan="2">Monday</th>
<th scope="col">Tuesday</th>
<th scope="col">Wednesday</th>
</tr>
</thead>
<tbody>
<tr>
<td>9:00 - 10:30</td>
<td>Web Development</td>
<td rowspan="2">Lab Session</td>
<td>Algorithms</td>
<td>Mathematics</td>
</tr>
<tr>
<td>11:00 - 12:30</td>
<td>Database Systems</td>
<td>Operating Systems</td>
<td>Networking</td>
</tr>
<tr>
<td>1:30 - 3:00</td>
<td colspan="4">Lunch Break & Self-Study</td>
</tr>
<tr>
<td>3:00 - 4:30</td>
<td>AI Fundamentals</td>
<td>Cyber Security</td>
<td>Project Work</td>
<td>Seminar</td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
Output Results
Time | Monday | Tuesday | Wednesday | |
---|---|---|---|---|
9:00 – 10:30 | Web Development | Lab Session | Algorithms | Mathematics |
11:00 – 12:30 | Database Systems | Operating Systems | Networking | |
1:30 – 3:00 | Lunch Break & Self-Study | |||
3:00 – 4:30 | AI Fundamentals | Cyber Security | Project Work | Seminar |